1対Nの高速な顔認証をFaceNetとFaissを使って実装する方法|PyTorch
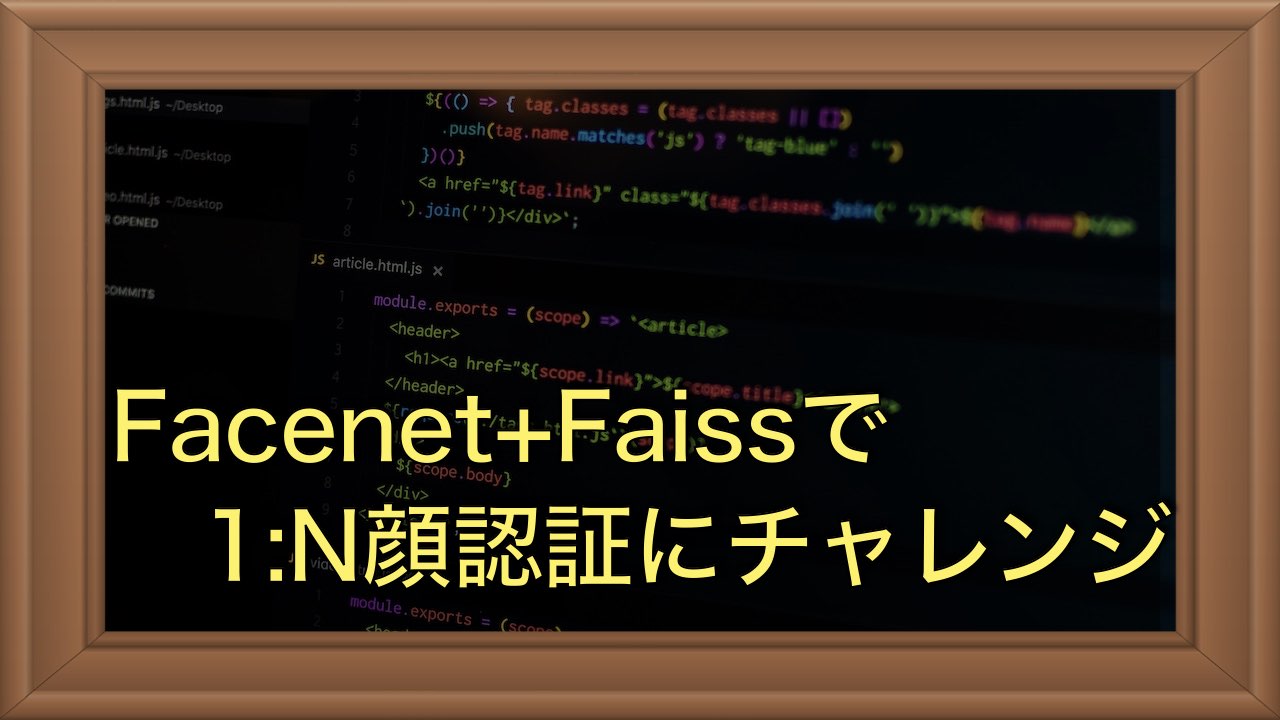
この記事では、FaceNetにFaissを組み合わせて、高速な1:N顔認証システムの構築にチャレンジします。このシステムでは、FaceNetで顔特徴量を抽出し、Faissで登録された顔との高速な照合を行います。これらのオープンソースを利用することで、手軽に高性能な顔認証システムを構築することが可能です。本記事では、実際のコードを交えながら、初めて顔認証に取り組む方にもわかりやすいように解説しています。
FaceNetを使って1:1の顔認証をする記事はこちら
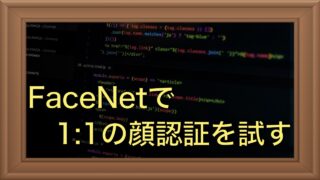
はじめに
これまで、MNISTの手書き数字識別を使って、以下のような実験を行ってきました。
- Pytorchで手書き文字認識
- Pytorch Metric Learningを使った深層距離学習とFaissを使ったベクトル検索
上記の2つの実験で、モデルの作成、深層距離学習による特徴量のベクトル化と、ベクトル化したデータの検索方法について確認できましたので、次は顔認証にトライしてみます。
顔認証を行うためには、顔特徴量を学習したモデルが必要になります。この学習は大変なので、ここではfacenetという学習済みモデルが用意されている顔認識(Face Recognition)ライブラリを利用しました。
また、実験に使う顔画像ですが、こちらは利用できそうなデータセットがkaggle上にあったので、それを利用しています。
データセットがkaggle上なので、ノートブックもkaggle notebookを使いました。
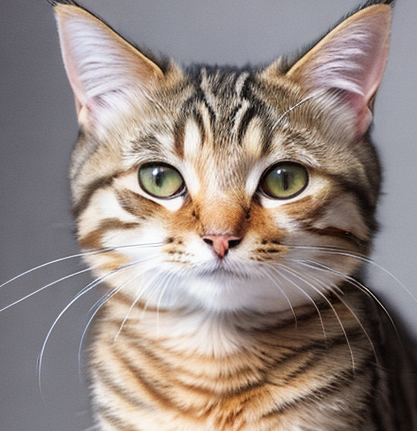
kaggle上で完結させると、データのコピーなどが必要なく楽なのでkaggle notebookを利用しました。
今回の記事で作成したノートブックなどのURLは以下の通りです(kaggleのデータセット、ノートブックなどへのアクセスにはkaggleのアカウントが必要かもしれません)。
Face Recognition Dataset : 顔画像のデータセット
https://www.kaggle.com/datasets/vasukipatel/face-recognition-dataset
facenet_pytorch+faiss.ipynb: 今回の記事のために作成したノートブック
https://www.kaggle.com/code/aruaru0/facenet-pytorch-faiss-ipynb/notebook
facenet_pytorch
facenet-pytorch
は、PyTorchを使用した顔認識(Face Recognition)ライブラリです。
このライブラリを使うことで、以下の処理を行うことが可能です。
- 顔の検出と抽出
画像から顔を検出し、それらの顔を抽出します。このプロセスは、顔の位置や姿勢の変化に頑健であり、様々な角度や照明条件で動作します。 - 顔の特徴量の計算
各顔に対して、顔の特徴を数値ベクトルとして抽出します。これらの特徴ベクトルは、顔の特徴をより抽象的に表現し、類似度の計算や識別に使用されます。
つまり、入力された画像から顔を抽出し、特徴量を計算する部分までをこのライブラリだけで行うことが可能です。
また、学習済みモデルも提供されているので、とりあえず学習なしで顔特徴量の抽出を行うことが可能です。
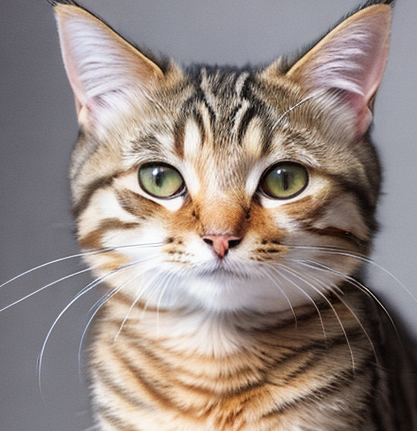
精度を上げたいなら、ファインチューニングした方が良いと思います。ファインチューニングの方法は、公式ページにあります
Github: https://github.com/timesler/facenet-pytorch
Faiss
Faiss
(Facebook AI Similarity Search)は、類似性検索(similarity search)のためのライブラリです。このライブラリを使うことで、大規模なデータのベクトル間の類似性を高速に計算することが可能です。
Faissの主な機能と特徴は以下の通りです
- 類似性検索の高速化
- GPUサポート
- 多様な類似性メトリクス(L2距離、内積などをサポート)
- メモリ効率の最適化
今回は、Faissをfacenetで得られた特徴ベクトルの検索に利用します
処理の流れとしては、①facenetで顔特徴量を抽出し、②faissで検索することで顔認証に挑戦してみます。
Faissをテキスト検索に使う方法については、以下の記事を参考にしてください

1対N 顔認証とは
顔認証には、1:1認証と1:N認証の2種類があります。
1対1認証とは、照合する本人の特徴ベクトルとの比較を行う方式です。特定の人物との照合だけを行うため、照合する処理が軽くなります。
一方、1対N認証は、登録されている多数の特徴ベクトルの中から合致する1人を選ぶという方式です。多数の人数との照合が必要となるため、処理が重くなります。
今回は1:N認証をFaissをつかって実装します。
顔認証を実装する
顔認証の実装の説明は、こちらのkaggleのノートブックの内容になります。
なお、顔画像のデータセットは、/kaggle/input/face-recognition-dataset/
にリンクされているとします(下図
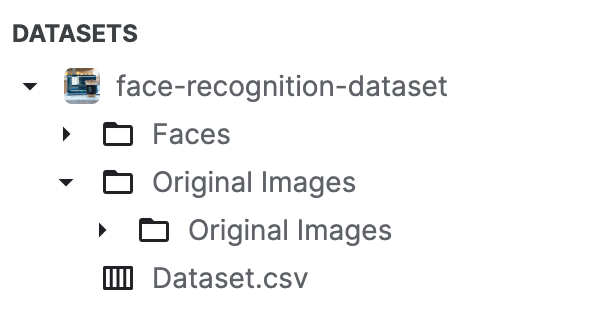
パッケージのインストール
必要なパッケージをインストールします。ノートブックの場合は!
をつけてセルを実行することでpipコマンドを実行できます。
なお、faiss-gpu
をインストールしていますが、CPUで動かす場合にはfaiss-cpu
をインストールします。
!pip install facenet_pytorch
!pip install faiss-gpu
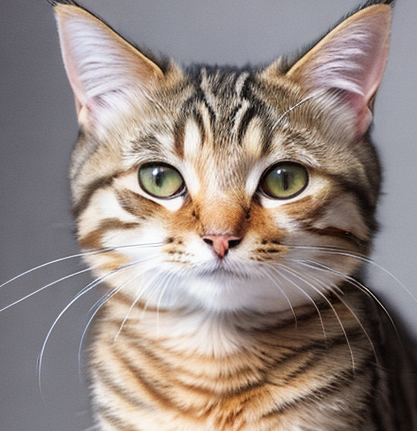
インストールは、ネットワークへの接続が許可されている必要があります。
ライブラリのインポート
今回のコードで必要となるライブラリをインポートします。
from facenet_pytorch import MTCNN, InceptionResnetV1
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
import faiss
import torch
import glob
from tqdm.notebook import tqdm
import random
顔画像を読み込む
顔画像を読み込みます。データセットには顔部分を切り出した画像と、元の画像(Original Image)が用意されていますが、今回は切り出し前の元画像を利用します。
フォルダを検索
フォルダを検索して、画像ファイルの一覧をfiles
に格納します。
files = glob.glob("/kaggle/input/face-recognition-dataset/Original Images/Original Images/*/*")
名前を抽出
ファイル名は、xx/name_num.jpg
のようになっているので、ここからname
部分を取り出します。/
で分割した一番後ろの文字列を_
で区切った先頭部分がname
になります。
labels = []
for file in files:
s = file.split("/")[-1].split("_")[0]
labels.append(s)
名前→ID、ID→名前の辞書を作成
Faissに番号で登録したいので、名前→ID番号に変換する辞書と、ID番号→名前に変換する辞書を作成します。
id2name = dict()
for i,e in enumerate(set(labels)):
id2name[i] = e
name2id = {id2name[i]:i for i in id2name}
DBに登録する画像を抽出
Faissで登録するデータ(train)と、登録しないデータ(test)に分割します。今回は、0番はじまりで偶数番目のデータを登録するデータ、奇数番目のデータを登録しないデータにしました。
また、train_labels, test_labelsに、各画像のID番号(正解)を格納しています。
train_files, train_labels = [],[]
for i in range(0, len(files), 2):
train_files.append(files[i])
train_labels.append(name2id[labels[i]])
test_files, test_labels = [], []
for i in range(1, len(files), 2):
test_files.append(files[i])
test_labels.append(name2id[labels[i]])
facenet, faissの初期化
device
にcudaまたはcpuを設定します
device = "cuda" if torch.cuda.is_available() else "cpu"
Faissの初期化を行います。今回は、量子化版を利用します。なお、登録人数に対して画像データ数が少ないためnbits=8
などとnbits
を大きくするとtrain
でワーニングが出ます。
dim = 512
nlist = 10
m = 32
nbits = 5
quantizer = faiss.IndexFlatIP(dim)
index = faiss.IndexIVFPQ(quantizer, dim, nlist, m, nbits, faiss.METRIC_INNER_PRODUCT)
facenetの顔検出を行うMTCNNと、顔特徴量を抽出するInceptionResnetV1を初期化します。
mtcnn = MTCNN(image_size=160, margin=10, device=device)
resnet = InceptionResnetV1(pretrained='vggface2').to(device).eval()
登録
Faissに登録を行うために、train_files
の画像の特徴ベクトルを計算します。計算した、ベクトルはembeddings
に格納します。
embeddings = None
for file in tqdm(train_files):
img = Image.open(file)
img = mtcnn(img).to(device)
embedding = resnet(img.unsqueeze(0)).cpu().detach().numpy()
if embeddings is None:
embeddings = embedding
else:
embeddings = np.concatenate((embeddings, embedding), axis=0)
embeddings
に格納したデータで特徴量の分布を学習し(index.train
)、データベースに追加します。これで、登録は完了です。
# 圧縮のために一部のデータで特徴量の分布を学習させる
index.train(embeddings)
index.add_with_ids(embeddings, np.array(train_labels))
登録したインデックスを保存したい場合には以下のコードを実行します
faiss.write_index(index, "xxxx.index")
また、読み込む場合には、以下のコードを実行します
faiss.read_index("xxxx.index")
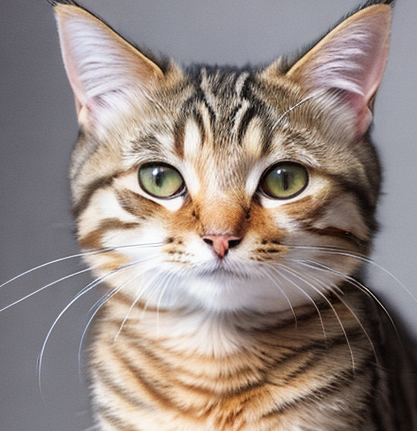
1:N認証にチャレンジ
いよいよ1:N認証にチャレンジしてみます。今回は、testデータのうち50枚に1枚だけ認証を行ってみます。
コードは以下になります。
- 顔を抽出(
mtcnn
) - 顔画像を特徴ベクトルに変換(
resnet
) - 特徴ベクトルでTOP10を検索(
index.search
) - 結果の表示
for i, (label, file) in enumerate(zip(test_labels, test_files)) :
if i%50 == 0 :
img = Image.open(file)
img = mtcnn(img).to(device)
embedding = resnet(img.unsqueeze(0)).cpu().detach().numpy()
D, I = index.search(embedding, 10)
print("label=", label, id2name[label])
for d, i in zip(D[0], I[0]):
if label == i :
print(" distance = ", d, "index = ", i, id2name[label], "---OK")
else:
print(" distance = ", d, "index = ", i, id2name[label], "xxxNG")
以下が実行結果になります。実行結果を見ると、すべての画像に対して、TOP10に本人の画像が選択されたことがわかります。今回は登録人数が少ないというのはありますが、特にチューニングなどしていないことを考えると、なかなかの認証精度ではないでしょうか。
また、IndexIVFPQ
を利用しているので検索時間も高速だと思います。仮に自作したとすると、1281枚x50枚の512ベクトルのコサイン類似度の計算量になりますが、それと比べると高速(?)に感じます。
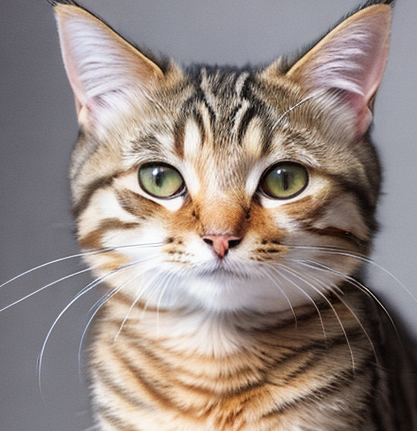
100万枚くらい登録すると差が顕著だと思いますが、この程度では愚直に計算しても検索速度の差を感じないかもしれません。
正直、NGが1つくらいでたほうが楽しかったのですが・・・
実行結果
label= 27 Alia Bhatt
distance = 0.75660205 index = 27 Alia Bhatt ---OK
distance = 0.7533354 index = 27 Alia Bhatt ---OK
distance = 0.74903935 index = 27 Alia Bhatt ---OK
distance = 0.74846125 index = 27 Alia Bhatt ---OK
distance = 0.74641603 index = 27 Alia Bhatt ---OK
distance = 0.7452564 index = 27 Alia Bhatt ---OK
distance = 0.744335 index = 27 Alia Bhatt ---OK
distance = 0.7432446 index = 27 Alia Bhatt ---OK
distance = 0.7429465 index = 27 Alia Bhatt ---OK
distance = 0.74200004 index = 27 Alia Bhatt ---OK
label= 4 Charlize Theron
distance = 0.6804388 index = 4 Charlize Theron ---OK
distance = 0.675962 index = 4 Charlize Theron ---OK
distance = 0.6752498 index = 4 Charlize Theron ---OK
distance = 0.67379314 index = 4 Charlize Theron ---OK
distance = 0.6722882 index = 4 Charlize Theron ---OK
distance = 0.6720895 index = 4 Charlize Theron ---OK
distance = 0.67131513 index = 4 Charlize Theron ---OK
distance = 0.6712791 index = 4 Charlize Theron ---OK
distance = 0.6700915 index = 4 Charlize Theron ---OK
distance = 0.66890347 index = 4 Charlize Theron ---OK
label= 6 Zac Efron
distance = 0.6859 index = 6 Zac Efron ---OK
distance = 0.6859 index = 6 Zac Efron ---OK
distance = 0.6859 index = 6 Zac Efron ---OK
distance = 0.6859 index = 6 Zac Efron ---OK
distance = 0.6859 index = 6 Zac Efron ---OK
distance = 0.6859 index = 6 Zac Efron ---OK
distance = 0.6859 index = 6 Zac Efron ---OK
distance = 0.6859 index = 6 Zac Efron ---OK
distance = 0.6859 index = 6 Zac Efron ---OK
distance = 0.6859 index = 6 Zac Efron ---OK
label= 29 Billie Eilish
distance = 0.794356 index = 29 Billie Eilish ---OK
distance = 0.7920514 index = 29 Billie Eilish ---OK
distance = 0.7896281 index = 29 Billie Eilish ---OK
distance = 0.7864181 index = 29 Billie Eilish ---OK
distance = 0.7822334 index = 29 Billie Eilish ---OK
distance = 0.7822334 index = 29 Billie Eilish ---OK
distance = 0.7822334 index = 29 Billie Eilish ---OK
distance = 0.7822334 index = 29 Billie Eilish ---OK
distance = 0.78168315 index = 29 Billie Eilish ---OK
distance = 0.7787674 index = 29 Billie Eilish ---OK
label= 15 Jessica Alba
distance = 0.796047 index = 15 Jessica Alba ---OK
distance = 0.796047 index = 15 Jessica Alba ---OK
distance = 0.796047 index = 15 Jessica Alba ---OK
distance = 0.796047 index = 15 Jessica Alba ---OK
distance = 0.796047 index = 15 Jessica Alba ---OK
distance = 0.79597664 index = 15 Jessica Alba ---OK
distance = 0.79597664 index = 15 Jessica Alba ---OK
distance = 0.7946643 index = 15 Jessica Alba ---OK
distance = 0.7942629 index = 15 Jessica Alba ---OK
distance = 0.7913149 index = 15 Jessica Alba ---OK
label= 8 Priyanka Chopra
distance = 0.79211754 index = 8 Priyanka Chopra ---OK
distance = 0.7914661 index = 8 Priyanka Chopra ---OK
distance = 0.79104507 index = 8 Priyanka Chopra ---OK
distance = 0.7909175 index = 8 Priyanka Chopra ---OK
distance = 0.79068893 index = 8 Priyanka Chopra ---OK
distance = 0.7897552 index = 8 Priyanka Chopra ---OK
distance = 0.7893305 index = 8 Priyanka Chopra ---OK
distance = 0.78895587 index = 8 Priyanka Chopra ---OK
distance = 0.78741926 index = 8 Priyanka Chopra ---OK
distance = 0.78679013 index = 8 Priyanka Chopra ---OK
label= 20 Natalie Portman
distance = 0.8161381 index = 20 Natalie Portman ---OK
distance = 0.80680746 index = 20 Natalie Portman ---OK
distance = 0.8057316 index = 20 Natalie Portman ---OK
distance = 0.80067873 index = 20 Natalie Portman ---OK
distance = 0.8006168 index = 20 Natalie Portman ---OK
distance = 0.8006168 index = 20 Natalie Portman ---OK
distance = 0.8006168 index = 20 Natalie Portman ---OK
distance = 0.79980254 index = 20 Natalie Portman ---OK
distance = 0.799388 index = 20 Natalie Portman ---OK
distance = 0.799388 index = 20 Natalie Portman ---OK
label= 0 Hrithik Roshan
distance = 0.7797378 index = 0 Hrithik Roshan ---OK
distance = 0.7776022 index = 0 Hrithik Roshan ---OK
distance = 0.77391076 index = 0 Hrithik Roshan ---OK
distance = 0.77008384 index = 0 Hrithik Roshan ---OK
distance = 0.7687974 index = 0 Hrithik Roshan ---OK
distance = 0.7686351 index = 0 Hrithik Roshan ---OK
distance = 0.76791275 index = 0 Hrithik Roshan ---OK
distance = 0.7678262 index = 0 Hrithik Roshan ---OK
distance = 0.76679367 index = 0 Hrithik Roshan ---OK
distance = 0.7661423 index = 0 Hrithik Roshan ---OK
label= 9 Tom Cruise
distance = 0.8050973 index = 9 Tom Cruise ---OK
distance = 0.8050973 index = 9 Tom Cruise ---OK
distance = 0.8050973 index = 9 Tom Cruise ---OK
distance = 0.8050973 index = 9 Tom Cruise ---OK
distance = 0.8050973 index = 9 Tom Cruise ---OK
distance = 0.8050973 index = 9 Tom Cruise ---OK
distance = 0.8050973 index = 9 Tom Cruise ---OK
distance = 0.80200076 index = 9 Tom Cruise ---OK
distance = 0.80031043 index = 9 Tom Cruise ---OK
distance = 0.80031043 index = 9 Tom Cruise ---OK
label= 7 Henry Cavill
distance = 0.7307897 index = 7 Henry Cavill ---OK
distance = 0.72912955 index = 7 Henry Cavill ---OK
distance = 0.72526413 index = 7 Henry Cavill ---OK
distance = 0.7193464 index = 7 Henry Cavill ---OK
distance = 0.71700823 index = 7 Henry Cavill ---OK
distance = 0.71601045 index = 7 Henry Cavill ---OK
distance = 0.709856 index = 7 Henry Cavill ---OK
distance = 0.7096555 index = 7 Henry Cavill ---OK
distance = 0.7094081 index = 7 Henry Cavill ---OK
distance = 0.70741194 index = 7 Henry Cavill ---OK
label= 7 Henry Cavill
distance = 0.7057308 index = 7 Henry Cavill ---OK
distance = 0.6933795 index = 7 Henry Cavill ---OK
distance = 0.69107896 index = 7 Henry Cavill ---OK
distance = 0.6846564 index = 7 Henry Cavill ---OK
distance = 0.6846081 index = 7 Henry Cavill ---OK
distance = 0.6843866 index = 7 Henry Cavill ---OK
distance = 0.6829125 index = 7 Henry Cavill ---OK
distance = 0.6814902 index = 7 Henry Cavill ---OK
distance = 0.68096834 index = 7 Henry Cavill ---OK
distance = 0.6786971 index = 7 Henry Cavill ---OK
label= 19 Brad Pitt
distance = 0.6540397 index = 19 Brad Pitt ---OK
distance = 0.6486824 index = 19 Brad Pitt ---OK
distance = 0.64867187 index = 19 Brad Pitt ---OK
distance = 0.64676887 index = 19 Brad Pitt ---OK
distance = 0.64420104 index = 19 Brad Pitt ---OK
distance = 0.64413977 index = 19 Brad Pitt ---OK
distance = 0.64413977 index = 19 Brad Pitt ---OK
distance = 0.6438623 index = 19 Brad Pitt ---OK
distance = 0.64374447 index = 19 Brad Pitt ---OK
distance = 0.6422262 index = 19 Brad Pitt ---OK
label= 16 Dwayne Johnson
distance = 0.95952934 index = 16 Dwayne Johnson ---OK
distance = 0.954877 index = 16 Dwayne Johnson ---OK
distance = 0.9525008 index = 16 Dwayne Johnson ---OK
distance = 0.9473208 index = 16 Dwayne Johnson ---OK
distance = 0.943702 index = 16 Dwayne Johnson ---OK
distance = 0.943141 index = 16 Dwayne Johnson ---OK
distance = 0.9412516 index = 16 Dwayne Johnson ---OK
distance = 0.9400626 index = 16 Dwayne Johnson ---OK
distance = 0.939986 index = 16 Dwayne Johnson ---OK
distance = 0.93837464 index = 16 Dwayne Johnson ---OK
label= 1 Elizabeth Olsen
distance = 0.79863864 index = 1 Elizabeth Olsen ---OK
distance = 0.7965965 index = 1 Elizabeth Olsen ---OK
distance = 0.7895905 index = 1 Elizabeth Olsen ---OK
distance = 0.78626525 index = 1 Elizabeth Olsen ---OK
distance = 0.78454477 index = 1 Elizabeth Olsen ---OK
distance = 0.7841255 index = 1 Elizabeth Olsen ---OK
distance = 0.783917 index = 1 Elizabeth Olsen ---OK
distance = 0.783917 index = 1 Elizabeth Olsen ---OK
distance = 0.783917 index = 1 Elizabeth Olsen ---OK
distance = 0.783917 index = 1 Elizabeth Olsen ---OK
label= 2 Camila Cabello
distance = 0.75801504 index = 2 Camila Cabello ---OK
distance = 0.7557689 index = 2 Camila Cabello ---OK
distance = 0.7557689 index = 2 Camila Cabello ---OK
distance = 0.7557689 index = 2 Camila Cabello ---OK
distance = 0.7557689 index = 2 Camila Cabello ---OK
distance = 0.7557689 index = 2 Camila Cabello ---OK
distance = 0.7557689 index = 2 Camila Cabello ---OK
distance = 0.7557689 index = 2 Camila Cabello ---OK
distance = 0.7557689 index = 2 Camila Cabello ---OK
distance = 0.7557689 index = 2 Camila Cabello ---OK
label= 13 Vijay Deverakonda
distance = 0.83182 index = 13 Vijay Deverakonda ---OK
distance = 0.82972026 index = 13 Vijay Deverakonda ---OK
distance = 0.82874215 index = 13 Vijay Deverakonda ---OK
distance = 0.8271393 index = 13 Vijay Deverakonda ---OK
distance = 0.8271056 index = 13 Vijay Deverakonda ---OK
distance = 0.82652116 index = 13 Vijay Deverakonda ---OK
distance = 0.82526886 index = 13 Vijay Deverakonda ---OK
distance = 0.8240138 index = 13 Vijay Deverakonda ---OK
distance = 0.8240138 index = 13 Vijay Deverakonda ---OK
distance = 0.823886 index = 13 Vijay Deverakonda ---OK
label= 21 Courtney Cox
distance = 0.7908816 index = 21 Courtney Cox ---OK
distance = 0.7902851 index = 21 Courtney Cox ---OK
distance = 0.78882253 index = 21 Courtney Cox ---OK
distance = 0.78882253 index = 21 Courtney Cox ---OK
distance = 0.7868762 index = 21 Courtney Cox ---OK
distance = 0.78683454 index = 21 Courtney Cox ---OK
distance = 0.78571707 index = 21 Courtney Cox ---OK
distance = 0.78569204 index = 21 Courtney Cox ---OK
distance = 0.78566206 index = 21 Courtney Cox ---OK
distance = 0.78495604 index = 21 Courtney Cox ---OK
label= 30 Ellen Degeneres
distance = 0.787713 index = 30 Ellen Degeneres ---OK
distance = 0.7870037 index = 30 Ellen Degeneres ---OK
distance = 0.785948 index = 30 Ellen Degeneres ---OK
distance = 0.785948 index = 30 Ellen Degeneres ---OK
distance = 0.785948 index = 30 Ellen Degeneres ---OK
distance = 0.785948 index = 30 Ellen Degeneres ---OK
distance = 0.785948 index = 30 Ellen Degeneres ---OK
distance = 0.785948 index = 30 Ellen Degeneres ---OK
distance = 0.785948 index = 30 Ellen Degeneres ---OK
distance = 0.785948 index = 30 Ellen Degeneres ---OK
label= 17 Anushka Sharma
distance = 0.76522 index = 17 Anushka Sharma ---OK
distance = 0.75723445 index = 17 Anushka Sharma ---OK
distance = 0.75723445 index = 17 Anushka Sharma ---OK
distance = 0.75723445 index = 17 Anushka Sharma ---OK
distance = 0.75723445 index = 17 Anushka Sharma ---OK
distance = 0.75723445 index = 17 Anushka Sharma ---OK
distance = 0.75648046 index = 17 Anushka Sharma ---OK
distance = 0.75648046 index = 17 Anushka Sharma ---OK
distance = 0.75648046 index = 17 Anushka Sharma ---OK
distance = 0.75648046 index = 17 Anushka Sharma ---OK
label= 12 Lisa Kudrow
distance = 0.82537913 index = 12 Lisa Kudrow ---OK
distance = 0.82537913 index = 12 Lisa Kudrow ---OK
distance = 0.82537913 index = 12 Lisa Kudrow ---OK
distance = 0.82537913 index = 12 Lisa Kudrow ---OK
distance = 0.82537913 index = 12 Lisa Kudrow ---OK
distance = 0.82537913 index = 12 Lisa Kudrow ---OK
distance = 0.82537913 index = 12 Lisa Kudrow ---OK
distance = 0.82537913 index = 12 Lisa Kudrow ---OK
distance = 0.82537913 index = 12 Lisa Kudrow ---OK
distance = 0.82537913 index = 12 Lisa Kudrow ---OK
label= 3 Robert Downey Jr
distance = 0.71673816 index = 3 Robert Downey Jr ---OK
distance = 0.7101323 index = 3 Robert Downey Jr ---OK
distance = 0.7065399 index = 3 Robert Downey Jr ---OK
distance = 0.7034088 index = 3 Robert Downey Jr ---OK
distance = 0.69736916 index = 3 Robert Downey Jr ---OK
distance = 0.69447005 index = 3 Robert Downey Jr ---OK
distance = 0.68175256 index = 3 Robert Downey Jr ---OK
distance = 0.6808301 index = 3 Robert Downey Jr ---OK
distance = 0.67999464 index = 3 Robert Downey Jr ---OK
distance = 0.6799129 index = 3 Robert Downey Jr ---OK
label= 11 Virat Kohli
distance = 0.71856475 index = 11 Virat Kohli ---OK
distance = 0.71856475 index = 11 Virat Kohli ---OK
distance = 0.71856475 index = 11 Virat Kohli ---OK
distance = 0.71856475 index = 11 Virat Kohli ---OK
distance = 0.71856475 index = 11 Virat Kohli ---OK
distance = 0.71856475 index = 11 Virat Kohli ---OK
distance = 0.71856475 index = 11 Virat Kohli ---OK
distance = 0.71856475 index = 11 Virat Kohli ---OK
distance = 0.71856475 index = 11 Virat Kohli ---OK
distance = 0.71856475 index = 11 Virat Kohli ---OK
label= 24 Claire Holt
distance = 0.7167264 index = 24 Claire Holt ---OK
distance = 0.7135164 index = 24 Claire Holt ---OK
distance = 0.71014184 index = 24 Claire Holt ---OK
distance = 0.6991775 index = 24 Claire Holt ---OK
distance = 0.69654423 index = 24 Claire Holt ---OK
distance = 0.69316715 index = 24 Claire Holt ---OK
distance = 0.69071645 index = 24 Claire Holt ---OK
distance = 0.6893084 index = 24 Claire Holt ---OK
distance = 0.6876944 index = 24 Claire Holt ---OK
distance = 0.6856713 index = 24 Claire Holt ---OK
label= 18 Andy Samberg
distance = 0.7878568 index = 18 Andy Samberg ---OK
distance = 0.7870033 index = 18 Andy Samberg ---OK
distance = 0.7864406 index = 18 Andy Samberg ---OK
distance = 0.78612405 index = 18 Andy Samberg ---OK
distance = 0.78435874 index = 18 Andy Samberg ---OK
distance = 0.7841571 index = 18 Andy Samberg ---OK
distance = 0.78317434 index = 18 Andy Samberg ---OK
distance = 0.7829764 index = 18 Andy Samberg ---OK
distance = 0.7827409 index = 18 Andy Samberg ---OK
distance = 0.7816937 index = 18 Andy Samberg ---OK
label= 5 Akshay Kumar
distance = 0.6606576 index = 5 Akshay Kumar ---OK
distance = 0.65131426 index = 5 Akshay Kumar ---OK
distance = 0.65117097 index = 5 Akshay Kumar ---OK
distance = 0.64895624 index = 5 Akshay Kumar ---OK
distance = 0.64895624 index = 5 Akshay Kumar ---OK
distance = 0.64895624 index = 5 Akshay Kumar ---OK
distance = 0.64895624 index = 5 Akshay Kumar ---OK
distance = 0.64895624 index = 5 Akshay Kumar ---OK
distance = 0.64819866 index = 5 Akshay Kumar ---OK
distance = 0.6455843 index = 5 Akshay Kumar ---OK
label= 10 Hugh Jackman
distance = 0.7498063 index = 10 Hugh Jackman ---OK
distance = 0.74730515 index = 10 Hugh Jackman ---OK
distance = 0.74398196 index = 10 Hugh Jackman ---OK
distance = 0.74290633 index = 10 Hugh Jackman ---OK
distance = 0.7400661 index = 10 Hugh Jackman ---OK
distance = 0.7338712 index = 10 Hugh Jackman ---OK
distance = 0.73286885 index = 10 Hugh Jackman ---OK
distance = 0.7316556 index = 10 Hugh Jackman ---OK
distance = 0.72931594 index = 10 Hugh Jackman ---OK
distance = 0.72828406 index = 10 Hugh Jackman ---OK
まとめ
facenet + faissで1:N顔認証を行ってみました。実際に運用する場合は、ファインチューニングなどで苦労すると思いますが、オープンソースだけでとりあえず顔認証が作れるというはすごいことだと思います。